1.りんごと言われて思い浮かぶ、4つの属性を考えよう。その4つの属性をインスタンス変数に持つ、Appleクラスを定義しよう。
class Apple():
def __init__(self, color, weight, stem_length, circumference):
self.color = color
self.weight = weight
self.stem_length = stem_length
self.circumference = circumference
2.円を表す Circleクラスを定義しよう。そのクラスに、面積を計算して返すメソッド areaを持たせよう。面積の計算には、Pythonの組み込みモジュール
math の pi定数が使えます。次に、Circle オブジェクトを作って、area メ
ソッドを呼び出し、結果を出力しよう。
import math
class Circle():
def __init__(self, radius):
self.radius = radius
def calculate_area(self):
return self.radius ** 2 * math.pi
a_circle = Circle(4)
print(a_circle.calculate_area())
3.三角形を表す Triangle クラスを定義して、面積を返す area メソッドを持
たせよう。そして Triangle オブジェクトを作って、area メソッドを呼び出し
て、結果を出力しよう。
class Triangle():
def __init__(self, base, height):
self.base = base
self.height = height
def calculate_area(self):
return self.height * self.base / 2
a_triangle = Triangle(20, 30)
print(a_triangle.calculate_area())
4.六角形を表す Hexagon クラスを定義しよう。そのクラスには、外周の長さを
計算して返すメソ ッド calculate_perimeter を定義しよう。そして、
Hexagon オブジェクトを作って calculate_perimeter メソッドを呼び出
し、結果を出力しよう。
class Hexagon():
def __init__(self, s1, s2, s3, s4, s5, s6):
self.s1 = s1
self.s2 = s2
self.s3 = s3
self.s4 = s4
self.s5 = s5
self.s6 = s6
def calculate_perimeter(self):
return self.s1 + self.s2 + self.s3 + self.s4 + self.s5 + self.s6
a_hexagon = Hexagon(1, 2, 3, 4, 5, 6)
print(a_hexagon.calculate_perimeter())
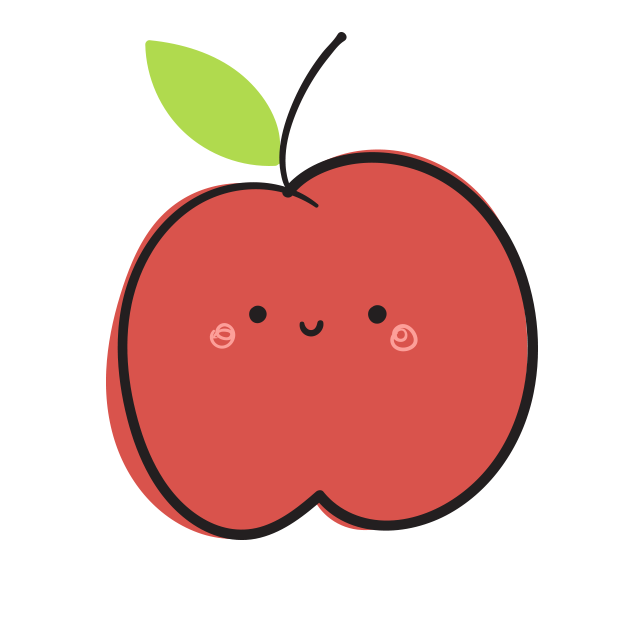
Apple Rooms laboratoryの運営者