if文とswitch文の復習
クリックした場所に円などの図形を描いてみよう
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>シンプルスタンプ</title>
<script>
let canvas,context; //キャンバス
const r = 60;
const init = () => {
//キャンバスの取得
canvas = document.getElementById("canvas");
context = canvas.getContext("2d");
}
const draw = event => {
//マウスカーソル位置の取得
const rect = event.target.getBoundingClientRect();
const x = event.clientX - rect.left;
const y = event.clientY - rect.top;
//スタンプを描画
const shape = document.getElementById("shape").value;
context.fillStyle = document.getElementById("color").value;
if (shape == "circle") {
//円を描画
context.beginPath();
context.arc(x,y,r,0,Math.PI*2);
context.fill();
} else if (shape == "rect") {
//正方形を描画
context.fillRect(x-r,y-r,r*2,r*2);
} else if (shape == "triangle") {
//三角形を描画
drawPolygon(3,x,y);
} else if (shape == "pentagon") {
//五角形を描画
drawPolygon(5,x,y);
}
}
const drawPolygon = (n,x,y) => {
//正n角形の描画
context.beginPath();
for (let i=0; i<n; i++) {
const angle = i/n * Math.PI*2 - Math.PI/2;
const tx = x + r * Math.cos(angle);
const ty = y + r * Math.sin(angle);
if (i==0) {
context.moveTo(tx,ty);
} else {
context.lineTo(tx,ty);
}
}
context.fill();
}
const clearCanvas = () => {
//キャンバスのクリア
context.clearRect(0,0,canvas.width,canvas.height);
}
</script>
<style>
canvas {border: thin solid #000000;}
</style>
<body onload="init()">
<p>シンプルスタンプ</p>
<input type="button" value="クリア" onclick="clearCanvas()">
スタンプの種類:<select id="shape">
<option value="circle">円
<option value="rect">正方形
<option value="triangle">三角形
<option value="pentagon">ご角形
</select>
色:<input type="color" id="color" value="#6699FF">
<hr>
<canvas id="canvas" width="800" height="600" onclick="draw(event)"></canvas>
</body>
</html>
</body>
http://ringo-apple-web.com/html/simple_stamp1.html
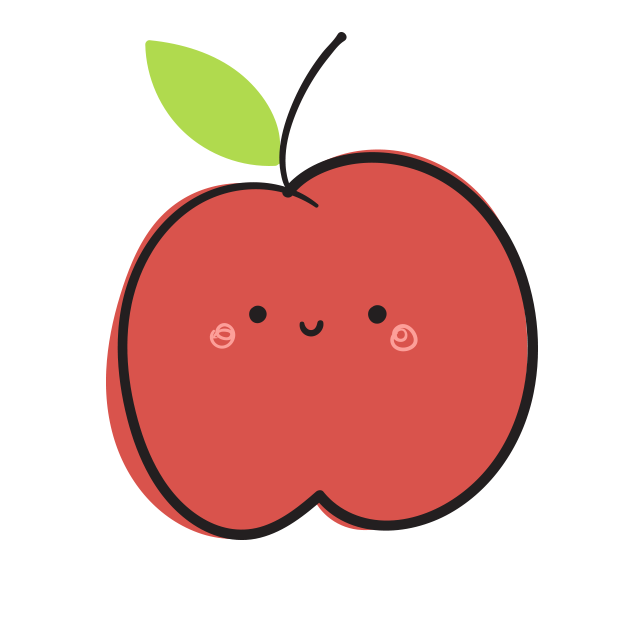
Apple Rooms laboratoryの運営者